A Comprehensive Guide on Sending SMS in Python
In the ever-evolving landscape of programming, Python stands out as a versatile language capable of handling various tasks. One such task that developers frequently encounter is sending SMS messages using Python. In this comprehensive guide, we will delve into the intricacies of sending SMS in Python, offering you a detailed walkthrough to empower your coding endeavors.
In this tutorial, we’ll show you how to use D7 Messaging API to send SMS messages from your Python application.
The code samples in this tutorial use the Direct7 Python package. Let’s get started!
Sign up for (or log in to) your D7 account
If you have a Verified D7 account with SMS capabilities, you’re all set! Feel free to jump straight to the code.
If you’re brand new to D7, you can sign up for a free trial account to get started. Once you've signed up, Claim your free credits with OTP verification and create a D7 application to get an API Token. You will need those values for the code samples below.
Now that you have a D7 application you can start sending messages to mobile devices.
Send an SMS message in Python via the REST API
To send an outgoing SMS message from your D7 account you’ll need to make an HTTP POST to D7.
Direct7 Python package helps you to create a new instance of the Message resource, specifying the recipients, content, originator, report_url, and Unicode parameters of your message.
If you don’t already have the Python package installed you can install it using pip:pip install direct7
Now, create a file named send_sms.py and include the following code:
from direct7 import Client
client = Client(api_token=”API token")
client.sms.send_message(recipients = ["+97150900XXXX","+97845900XXX"], content = "Greetings from D7 API", originator = "SignOTP", report_url = "https://the_url_to_recieve_delivery_report.com", unicode = False)
Replace the placeholder values for the API token with your unique values. You can find these in your API tokens in the d7 dashboard.
You’ll tell Direct7 which Sender/Header to use to send this message by replacing the originator. You can use your brand name with a maximum character limit of 11 or your mobile number with your country code. If you would like to register a new Sender ID, you can submit your details here.
You can also set the Default Originator here, and all your messages will be prefixed with the given originator.
Next, specify yourself as the message recipient by replacing the recipient's number with your mobile phone number. Both of these parameters must use a country code prefix (+ and a country code, e.g.,+16175551212). You can also set the Default Country here, and all your messages will be prefixed with the selected country code.
We also include the content parameter, which contains the content of the SMS we’re going to send. A long message over 160 characters will be split into multiple messages with 153 characters each and forwarded to telecom. When Unicode messages exceed 70 characters, they are split into multiple messages of 63 characters each. Also, billing will be based on the number of message parts sent.
You can also send normal text and Unicode messages by setting the parameter unicode as true or false. Set as false for normal GSM 03.38 characters(English, normal characters). Set as true for non-GSM 03.38 characters (Arabic, Chinese, Hebrew, Greek-like regional languages, and Unicode characters).
You can set the report_url parameter to receive delivery status (DLR) for your message, and specify the callback server URL where you want to receive the message status updates using the report_url parameter. When the delivery status changes, the status updates will be sent to the specified URL. For information on the format of the DLR message, please refer to the "Receiving DLR" section.
Once you’ve updated the code sample, you can test it out by running it from the command line: python send_sms.py
In just a few moments you should receive an SMS!
Let’s take a moment to understand what’s going on behind the scenes when you send this request to D7.
Direct7's response
When Direct7 receives your request to send an SMS via the REST API, it will check that you’ve included a valid API token. Direct7 will then either queue the SMS or return an HTTP error in its response to your request.
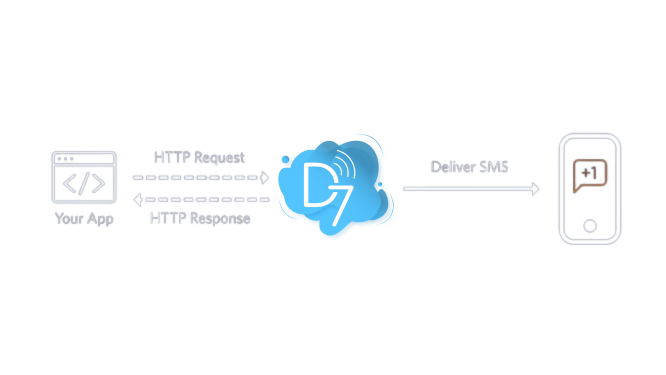
When the request is validated, request_id, status, and created time will be returned. A sample response might look like this
{ "request_id": "d9835609-a4e0-10ea-a26a-eeafbe700fef",
"status": "accepted",
"created_at": "2001-08-05T12:52:38.393Z"}
To see the status of your message, include the following code with the above request_id.
from direct7 import Client
client = Client(api_token="API token")
client. sms.get_status(request_id="request_id")
Best Practices for Sending SMS in Python
Now that you have a working code snippet, let's explore some best practices to enhance the effectiveness of your SMS-sending application.
Error Handling
Implement robust error handling to gracefully manage potential issues, such as network errors or D7 API failures.
Message Personalization
Personalize your messages to create a more engaging user experience. Include dynamic content and user-specific information to make your SMS stand out.
Rate Limiting
Be mindful of rate limiting imposed by D7 API to avoid disruptions. Adjust your sending frequency to comply with D7's guidelines.
In conclusion, sending SMS in Python can be a seamless process with the right tools and knowledge. By leveraging the power of the D7 library, you can incorporate SMS
functionality into your Python applications effortlessly. Remember to follow best practices, personalize your messages, and handle errors gracefully to create a reliable and user-friendly SMS-sending solution.
Leave your comments